Hacking Bath with Socrata
Blog post created on 2014-10-31
For how to get started with Socrata, skip the introduction. This weekend I will be undertaking my first ever hackathon. The event is hosted by Bath: Hacked and the teams will have up to 36 hours to create an app using "open data". All the data is local (to Bath) and is provided by the council. Bath: Hacked itself is a "joint council/community initiative that aims to put open data and smart thinking at the heart of the city". The data is hosted using Socrata's platform which, in this context, enables data producers to upload and publish data on the web. The platform enables users to interact with the data in several formats and also provides a simple query language for developers. To get familiar with this technology before the weekend I tried to build a simple client side application which I will explain here.
A Simple Tutorial
One of the simplest tasks you can achieve is probably plotting coordinates on a map so as a start you need to find a dataset which provides locations. For this exercise we will be using the BANES Live Car Park Occupancy dataset. The dataset provides a list of car parks in Bath. The application we will be building will be purely client side and therefore you won't need a server for testing purposes.
Create the HTML page
Open a text file in your favourite editor and save the file with a .html ending. I chose index.html. As we are only plotting points we shall create a full screen Google Maps page. Every HTML page needs the following tags as a bare minimum: html
, head
, body
and title
. We also need the doctype
at the top which in this case will be HTML5.
<!DOCTYPE html> <html> <head> <title>My First Socrata App</title> </head> <body></body> </html>
To have easier interaction with the map we will be using Google's Maps API which we will have to include in our head tag (Google is your friend).
Adding the Map
We need a single div on the page which will host the map. Interaction with the map will then be directly achieved with JavaScript. The div will have to be as high and as wide as the screen for a full screen experience. To ensure browser differences are at a minimum we also have to include a CSS reset file in our head tag. Our HTML body now becomes:
<body> <div id="map"></div> </body>
The required CSS is:
html, body, #map { width: 100%; height: 100%; }
We need to ensure both parent elements have a height specified when using percentages otherwise by default they all start with 0 height. Now onto the interesting part.
For simplicity we will be using jQuery. After including jQuery in the head of your page we need to add the map within our #map
div as soon as the page loads. The document ready callback is used for this purpose. Inside of our callback we can:
- Create the map and place it within our chosen div by passing the element to the map constructor;
- Center the map on Bath. We can find coordinates using Google;
- Set the zoom level, test and experiment to find the right one.
This is all done with the following JavaScript snippet:
$(document).ready(function() { var map = new google.maps.Map(document.getElementById("map"), { center: new google.maps.LatLng(51.380579, -2.360215), zoom: 13 }); });
Loading the Car Park Locations
Interacting with a Socrata dataset is easy. Once we have the ID of our dataset we can find the json format at the following URL: https://DATASTORE/resource/ID.json
with DATASTORE being the site currently hosting the datasets, in our case data.bathhacked.org. The ID
, which you can find when browsing the datasets, in our case is u3w2-9yme
.
All we are missing is to use jQuery's getJSON
function to fetch the json format of the data and add the markers to the map. Put simply we need to:
- Fetch the data;
- Loop over each entry;
- Create a marker with the coordinates taken from the entry;
- Add the marker to the map;
This can be done all within the loop and the constructor of the marker which themselves can be placed in the callback of the getJSON
function. The code is:
$.getJSON(url, function(data, textstatus) { $.each(data, function(i, entry) { var lat = entry.location.latitude; var lon = entry.location.longitude; var marker = new google.maps.Marker({ position: new google.maps.LatLng(lat, lon), map: map, title: entry.name }); }); });
We need to look at the column names of our data set to retrieve the right information. As you can see from the example above we are also adding a title label, by accessing our entry.name
value. This code needs to be placed right after we create the map, within the document ready callback.
At this point, if you open the file in a browser, you should have a working map like the one shown below:
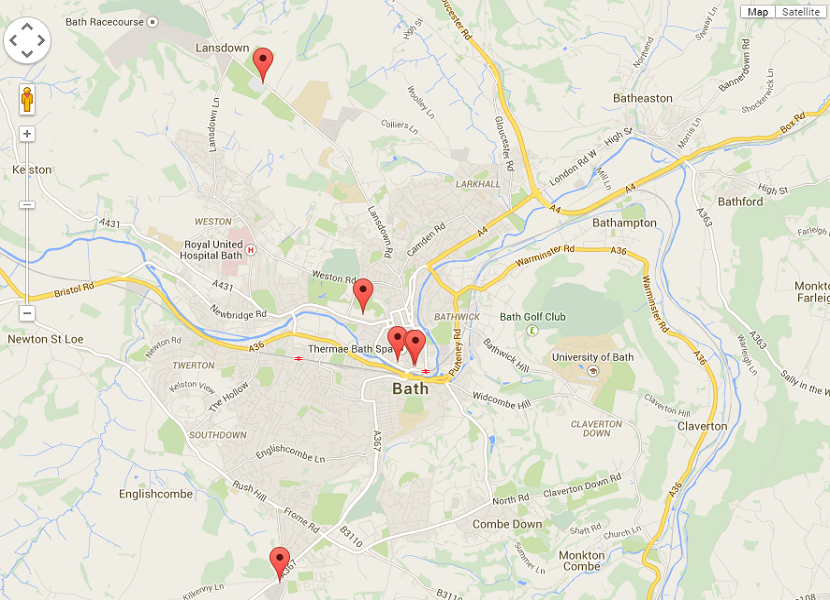
And that is your first Socrata application! Of course I went over the top a little and ended up building Bath Car Parks but that is another story. You can find the full source code in txt format for this post here.